I am using Visual Studio 2015, and I used the NuGet package manager to download and install JQuery. Below are a list of files installed. However I don't see the jquery-ui.js jQuery file anywhere? Has anyone else experienced this? Do I somehow have to manually install the UI code for JQuery? Jquery-1.12.3.js jquery-1.12.3.min.js jquery-1.12.3. Step 1: Launch Visual Studio Code Visual Studio Marketplace. Step 2: Go to extensions tab - Ctrl-Shift-X (Windows, Linux) or Cmd-Shift-X (OSX) Step 3: Search for 'mdbsnippets'. Step 4: Choose the extension. Step 5: Install extension and reload Visual Studio Code.
- Visual Studio Code Download
- Visual Studio Code Jquery Extension
- Run Jquery In Visual Studio Code
- Jquery Visual Studio Code
Before starting
If you really just want to execute the steps without any explanation, try to jump right to the Conclusion section.
That being said, you can always read the parts that you are not sure about later :)
Prerequisites
Before going further, you will need node.js
and npm
. If you don’t know what those are, lets just say that npm
is a package manager and it requires Node. We will use npm
from within VS Code (in command line), to help us install our jQuery “IntelliSense” definition file.
- NPM is packaged with Node, so no more action are required here :)

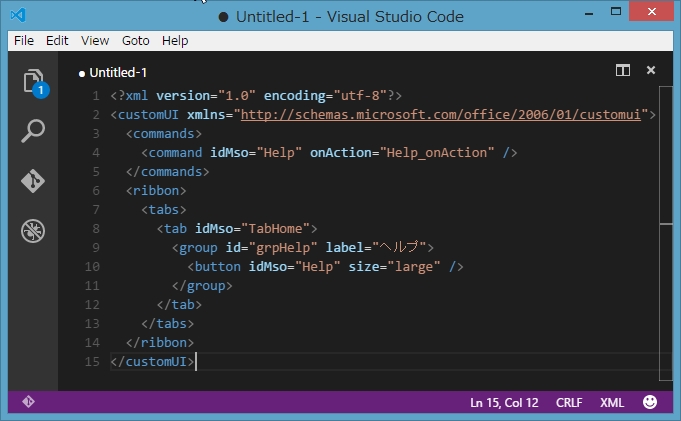
Install Node and NPM and make sure the path is added to you PATH
environment variable. This way, you will be able to get access to node and npm from everywhere without typing the file path every time. Which will become really useful really fast, I am telling you.
If I remember well, during the installation process, the installer will prompt you about that. But its been a while since I installed Node, so I might be wrong here.
After the installation, open a console (cmd
for Windows users) and type npm
. If this does not work, look at the following link: fixing npm path in Windows 8. For other Windows versions this will be pretty similar. For other OS, I really don’t know - Google is your friend tho… sry
jQuery IntelliSense
The project directory
Now that Node.js and NPM are installed, lets create a Visual Studio Code folder
. I created my project there at F:ReposBlogPostjquery-intellisense
.
Here is my project structure:
In my index.html
file I used the Bootstrap 3 Snippets extension to create the basic layout (bs3-template:html5
snippet). I changed the linked jQuery file to the version 3.1.0 and I also included the integrity
and crossorigin
attributes to my script
tag.
Here is the full index.html
file:
jQuery TypeScript type definitions
Now, you might think the following:
Why is the title saying JavaScript but now you talk about TypeScript ?
We will use a TypeScript type definitions file in JavaScript.
If you don’t know TypeScript, this is also a nice topic to look into. But lets keep this for another day…
Installing TSD globally
Lets install the npm package named TSD globally, by typing the following command:
Open a console (a VS Code terminal, a cmd
for Windows users, etc.), it doesn’t really matter since we are installing the package globally.
Installing the jQuery TypeScript type definitions file
Once this is done, open a new console (or use the same one), but this time, make sure that you are in your project folder
.
Type the following command:
This will install the TypeScript type definitions file we are looking for.
Your project structure should now look like this:
Use the definition file(s)
Lets open our app.js
file. If you type $
there is still nothing. To enable jQuery IntelliSense, we need to add a ///
reference instruction to our JavaScript file.
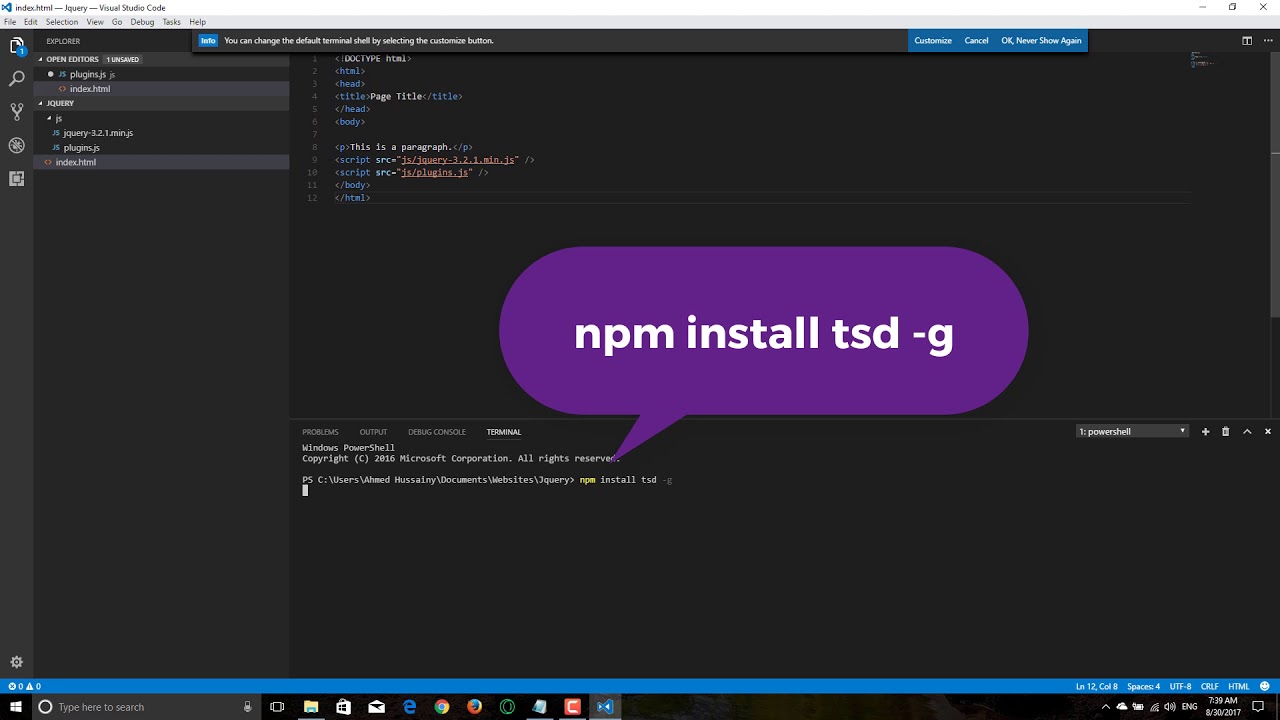
For some reasons, VS Code does not update after you type the path
so if you are stuck with an error like the following, reopen the file and it should fix it.
Here is the copyable snippet:
This basically told VS Code to append the type definitions contained in our tsd.d.ts
file to its actual IntelliSense.
If you open the tsd.d.ts
file you will see a reference to jquery/jquery.d.ts
which is our jQuery type definitions file (the file we “installed” earlier). So, by linking the tsd.d.ts
in our JavaScript files, it allows us to include all our loaded type definitions files at once (we only have jQuery for now, but who knows the future ;) ).
Back to our app.js
file, we now have full jQuery IntelliSense:
What to do in your next project
Now that we have everything setup and working, the only thing you need to do in your next project is to Install the jQuery TypeScript type definitions file and reference
it in your JavaScript files, as explained in the Use the definition file(s) section.
So the command you want to remember is this one:
And this one:
Note that the value of the path
attribute must match your tsd.d.ts
file location, so you might need to adjust it.
Conclusion
It was pretty strait forward, assuming you already had Node.js and npm installed, we did the following:
- Create a folder and add some file in it (or open an existing one)
- Install TSD globally using the following command:
npm install tsd -g
- Install the jQuery type definitions file in our project using the following command:
tsd install jquery --save
- Add a reference to our
tsd.d.ts
file in ourapp.js
file (or the file you want jQuery IntelliSense in) using the following triple-slash comment:/// <reference path='../typings/tsd.d.ts' />
… And voilà!
Happy coding!
Buy My Book Amazon.com (associate link)
Buy My Book Amazon.ca (associate link)
You liked the article
and think I deserve a
little something?
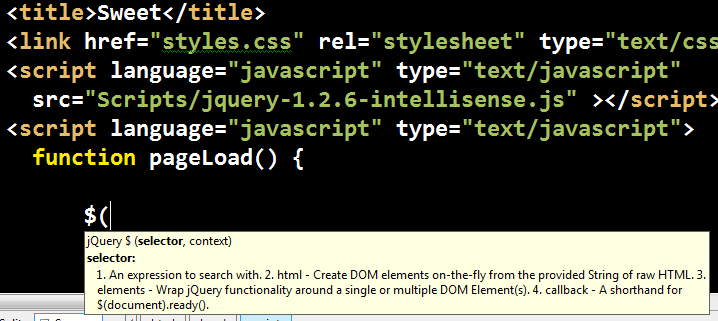
Visual Studio provides a powerful JavaScript editing experience right out of the box. Powered by a TypeScript based language service, Visual Studio delivers richer IntelliSense, support for modern JavaScript features, and improved productivity features such as Go to Definition, refactoring, and more.
Note
Starting in Visual Studio 2017, the JavaScript language service uses a new engine for the language service (called 'Salsa'). Details are included in this article, and you can also read this blog post. The new editing experience also mostly applies to Visual Studio Code. See the VS Code docs for more info.
For more information about the general IntelliSense functionality of Visual Studio, see Using IntelliSense.
What's new in the JavaScript language service in Visual Studio 2017
Starting in Visual Studio 2017, JavaScript IntelliSense displays a lot more information on parameter and member lists. This new information is provided by the TypeScript language service, which uses static analysis behind the scenes to better understand your code.
TypeScript uses several sources to build up this information:
Visual Studio Code Download
IntelliSense based on type inference
In JavaScript, most of the time there is no explicit type information available. Luckily, it is usually fairly easy to figure out a type given the surrounding code context.This process is called type inference.
For a variable or property, the type is typically the type of the value used to initialize it or the most recent value assignment.
For a function, the return type can be inferred from the return statements.
For function parameters, there is currently no inference, but there are ways to work around this using JSDoc or TypeScript .d.ts files (see later sections).
Additionally, there is special inference for the following:
- 'ES3-style' classes, specified using a constructor function and assignments to the prototype property.
- CommonJS-style module patterns, specified as property assignments on the
exports
object, or assignments to themodule.exports
property.
IntelliSense based on JSDoc
Where type inference does not provide the desired type information (or to support documentation), type information may be provided explicitly via JSDoc annotations. For example, to give a partially declared object a specific type, you can use the @type
tag as shown below:
As mentioned, function parameters are never inferred. However, using the JSDoc @param
tag you can add types to function parameters as well.
See JSDoc support in JavaScript for the JsDoc annotations currently supported.
IntelliSense based on TypeScript declaration files
Because JavaScript and TypeScript are now based on the same language service, they are able to interact in a richer way. For example, JavaScript IntelliSense can be provided for values declared in a .d.ts file (see TypeScript documentation), and types such as interfaces and classes declared in TypeScript are available for use as types in JsDoc comments.
Below, we show a simple example of a TypeScript definition file providing such type information (via an interface) to a JavaScript file in the same project (using a JsDoc
tag).
Automatic acquisition of type definitions
In the TypeScript world, most popular JavaScript libraries have their APIs described by .d.ts files, and the most common repository for such definitions is on DefinitelyTyped.
By default, the Salsa language service will try to detect which JavaScript libraries are in use and automatically download and reference the corresponding .d.ts file that describes the library in order to provide richer IntelliSense. The files are downloaded to a cache located under the user folder at %LOCALAPPDATA%MicrosoftTypeScript.
Visual Studio Code Jquery Extension
Note

Run Jquery In Visual Studio Code
This feature is disabled by default if using a tsconfig.json configuration file, but may be set to enabled as outlined further below.
Currently auto-detection works for dependencies downloaded from npm (by reading the package.json file), Bower (by reading the bower.json file), and for loose files in your project that match a list of roughly the top 400 most popular JavaScript libraries. For example, if you have jquery-1.10.min.js in your project, the file jquery.d.ts will be fetched and loaded in order to provide a better editing experience. This .d.ts file will have no impact on your project.
If you do not wish to use auto-acquisition, disable it by adding a configuration file as outlined below. You can still place definition files for use directly within your project manually.
Jquery Visual Studio Code
See also
